How to View Room Information and Join¶
Your player may need to view a list of matching rooms and choose one to join. Another similar case is that your player views information of a matching room and decide whether to join it. Examples include:
On a list of open instance dungeons (a room represents a dungeon)
Details of your squad that you have just randomly put into (a room represents a squad)
An invitation to a party received from a friend (a room represents a party)
Assuming you can get required information from room properties,
you need a Strix Room
or Strix Node Room
struct for the room
to show the room information to the player.
Also, after some interaction with them,
you will need to join the room (or one of the rooms).
Strix Node Room Info
struct will be most suitable,
because you can join the room directly with a Strix Node Room Info
struct,
and it has a Strix Node Room
struct for the room inside.
Note
If you are unfamiliar with room properties available on Strix Node and Strix Node Room structs, see Room Properties.
Showing a list of rooms¶
To show a list of open dungeons,
you usually use Search Node Room
function, giving appropriate set of conditions.
Success callback of Search Node Room
gives you an array of Strix Node Room Info
structs
(in an event parameter of name Node Rooms
),
from which you can get Strix Node Room
structs to show the room information,
or by which you can join the room.
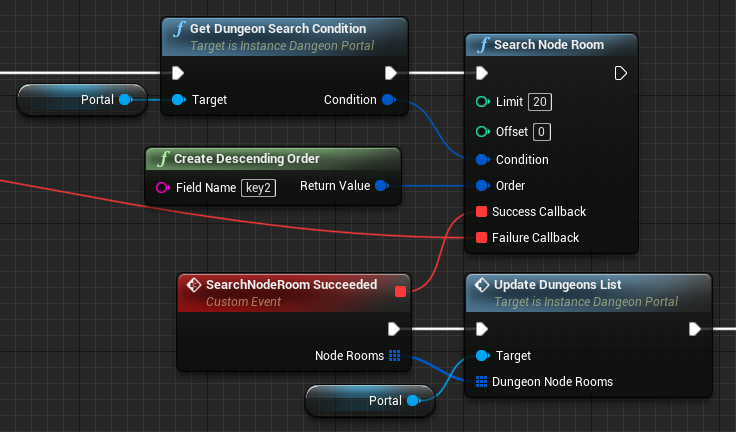
Getting the room you are currently in¶
If you are already in a room without specifying a particular room
(as a result of Join Random Node Room function, for example),
the Get Current Room
function returns a Strix Room
struct
corresponding to the current room.
You can use it to present the room information to the player.
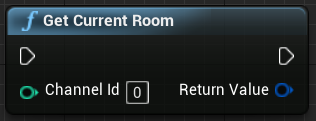
Getting a NodeRoom by an identifier¶
A common practice to invite (or instruct) another player to join a particular room
is to pass a unique identifier of the room.
You can use a primary key (primaryKey
property) of a NodeRoom
(i.e., Id
member variable of a Strix Node Room
struct) for the purpose.
Alternatively, you can use a primary key (primaryKey
property) of a room
(i.e., Id
member variable of a Strix Room
struct),
that is equivalent to a room ID (roomId
property) of a NodeRoom
(i.e., RoomId
member variable of a Strix Node Room
struct),
in combination with the address of the room server.
Note
primaryKey property of a room is unique within a single room server, but there may be another room with an identical primaryKey on another room server. If you have (or may have in the future) two or more room servers, you need to combine a room primary key with an identification of a room server to uniquely identify a room.
The client that received an identifier needs to find a Strix Node Room Info
struct
for the given identifier to show the room information to the player
and then to join the room later.
You need to use some C++ codes to do so.
There are three suggested techniques:
To receive a NodeRoom’s primary key then find and join the NodeRoom.
To receive a server address and a room’s primary key then find and join the NodeRoom.
To receive a server address and a room’s primary key then find and join the room.
Finding a NodeRoom by its primary key¶
If you receive a NodeRoom’s primary key as a unique identifier, you can search for a NodeRoom whose primary key matches the desired primary key. You need a helper function written in C++ to do so.
The helper function, called CreateStrixPrimaryKeyEqualsCondition
in C++ and primaryKey Field Equals
in Blueprint is as follows:
#pragma once
#include "CoreMinimal.h"
#include "Kismet/BlueprintFunctionLibrary.h"
#include "StrixBlueprintFunctionLibrary.h"
#include "MyBlueprintFunctionLibrary.generated.h"
UCLASS()
class MYPROJECT_API UMyBlueprintFunctionLibrary : public UBlueprintFunctionLibrary
{
GENERATED_BODY()
UFUNCTION(BlueprintPure, Category = "MyBPLibrary|Adapter", meta = (DisplayName = "primaryKey Field Equals"))
static FStrixCondition CreateStrixPrimaryKeyEqualsCondition(int32 primaryKeyValue);
};
#include "MyBlueprintFunctionLibrary.h"
#include <strix/net/object/ObjectAdapter.h>
#include <strix/client/core/model/manager/filter/builder/ConditionBuilder.h>
using namespace strix::net::object;
using namespace strix::client::core::model::manager::filter::builder;
FStrixCondition UMyBlueprintFunctionLibrary::CreateStrixPrimaryKeyEqualsCondition(int32 primaryKeyValue)
{
FStrixCondition condition(ConditionBuilder::Builder()
->Field("primaryKey")->EqualTo(ObjectAdapter((int64)primaryKeyValue))
->Build());
return condition;
}
Then, the following Blueprint script gets the desired NodeRoom.
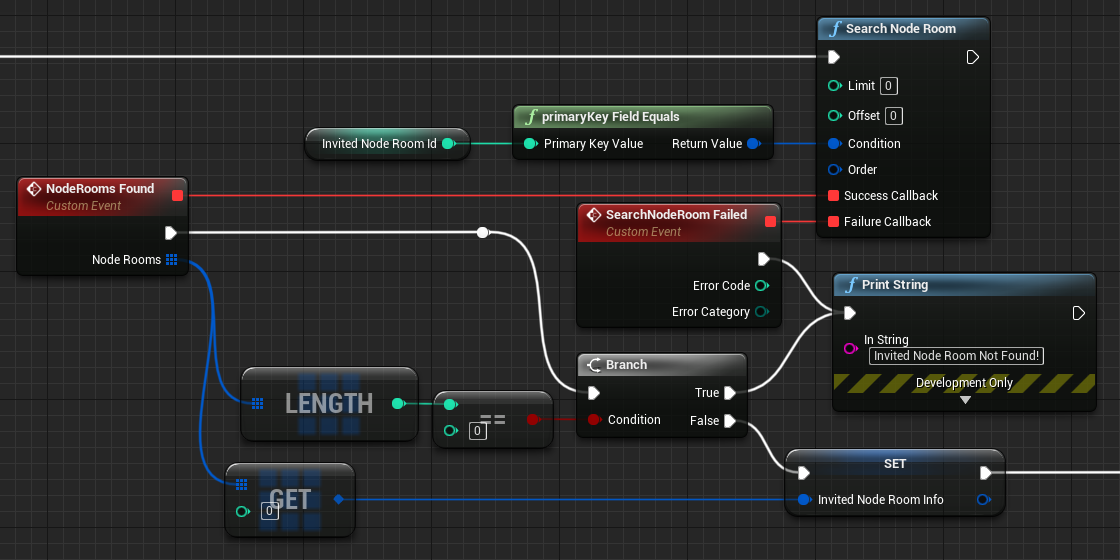
The Blueprint script above takes the variable Invited Node Room Id
as an input
and puts a Strix Node Room Info
struct for the room
in the variable Invited Node Room Info
when finished.
Finding a NodeRoom by its room id¶
If you receive a combination of a room id (room’s primary key) and a room server address (hostname, port, and protocol), you can search for a list of NodeRooms by roomId, then find one on the desired room server among them. (Remember that there may be two or more NodeRooms of same roomId on different room servers.) You need another helper function written in C++ for the purpose.
#pragma once
#include "CoreMinimal.h"
#include "Kismet/BlueprintFunctionLibrary.h"
#include "StrixBlueprintFunctionLibrary.h"
#include "MyBlueprintFunctionLibrary.generated.h"
UCLASS()
class MYPROJECT_API UMyBlueprintFunctionLibrary : public UBlueprintFunctionLibrary
{
GENERATED_BODY()
UFUNCTION(BlueprintPure, Category = "MyBPLibrary|Adapter", meta = (DisplayName = "roomId Field Equals"))
static FStrixCondition CreateStrixRoomIdEqualsCondition(int32 roomId);
};
#include "MyBlueprintFunctionLibrary.h"
#include <strix/net/object/ObjectAdapter.h>
#include <strix/client/core/model/manager/filter/builder/ConditionBuilder.h>
using namespace strix::net::object;
using namespace strix::client::core::model::manager::filter::builder;
FStrixCondition UMyBlueprintFunctionLibrary::CreateStrixRoomIdEqualsCondition(int32 roomId)
{
FStrixCondition condition(ConditionBuilder::Builder()
->Field("roomId")->EqualTo(ObjectAdapter((int64)roomId))
->Build());
return condition;
}
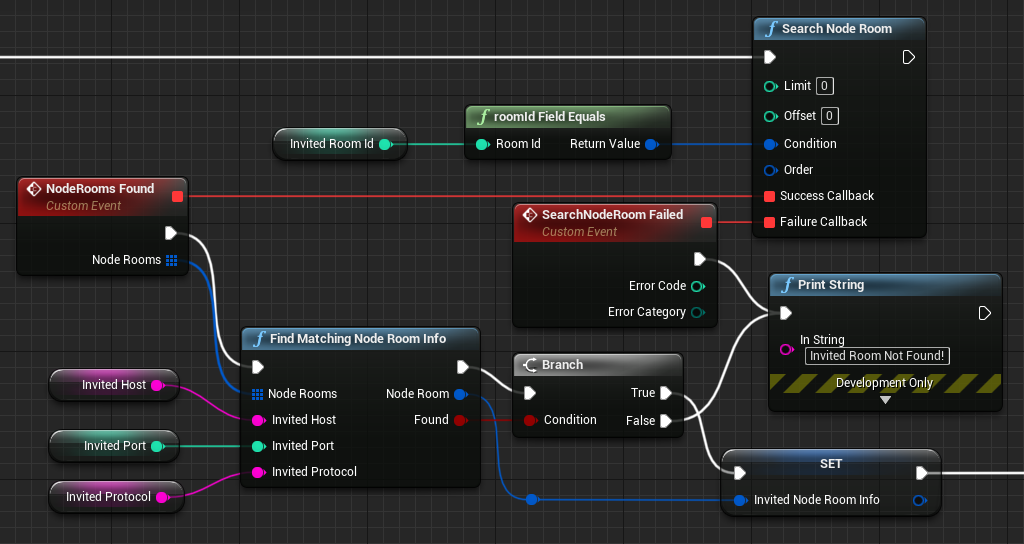
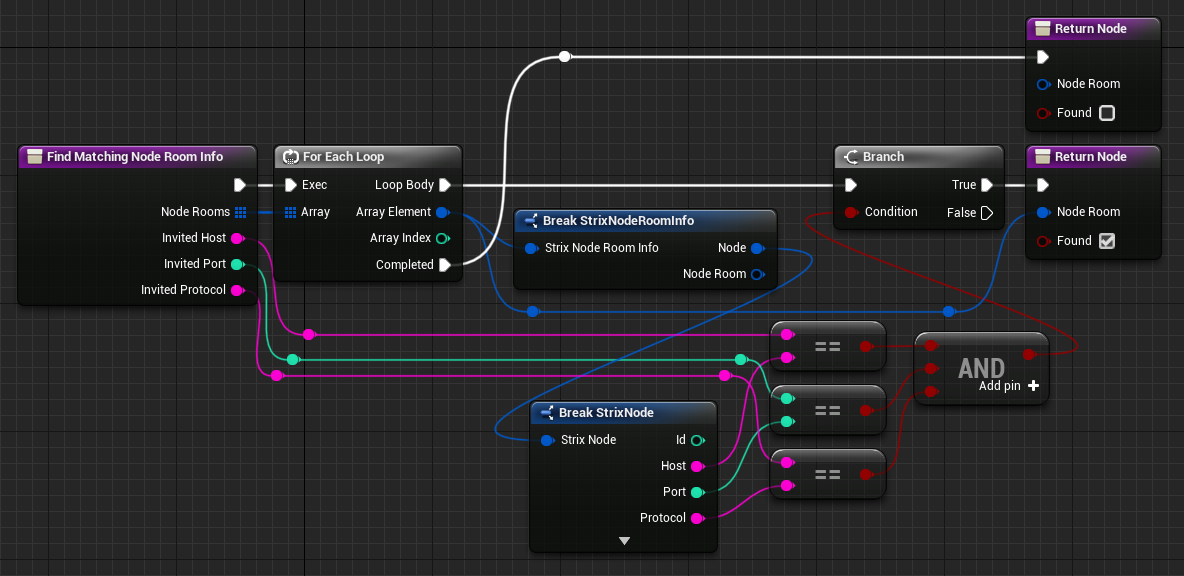
The Blueprint script above takes the variables
Invited Room Id
, Invited Host
, Invited Port
, and Invited Protocol
as inputs
and puts a Strix Node Room Info
struct in the variable Invited Node Room Info
when finished.
Finding a room by its room id¶
Finding a Strix Node Room Info
struct from a room id and a room server address
requires some steps as we see above.
It is easier to find a Strix Room
struct.
You can first connect to the room server using the received address
then call Search Room
to find the Strix Room
struct.
You can then join the room using the room ID on the same room server connection.
You need the CreateStrixPrimaryKeyEqualsCondition
C++ function (aka primaryKey Field Equals
)
explained above.
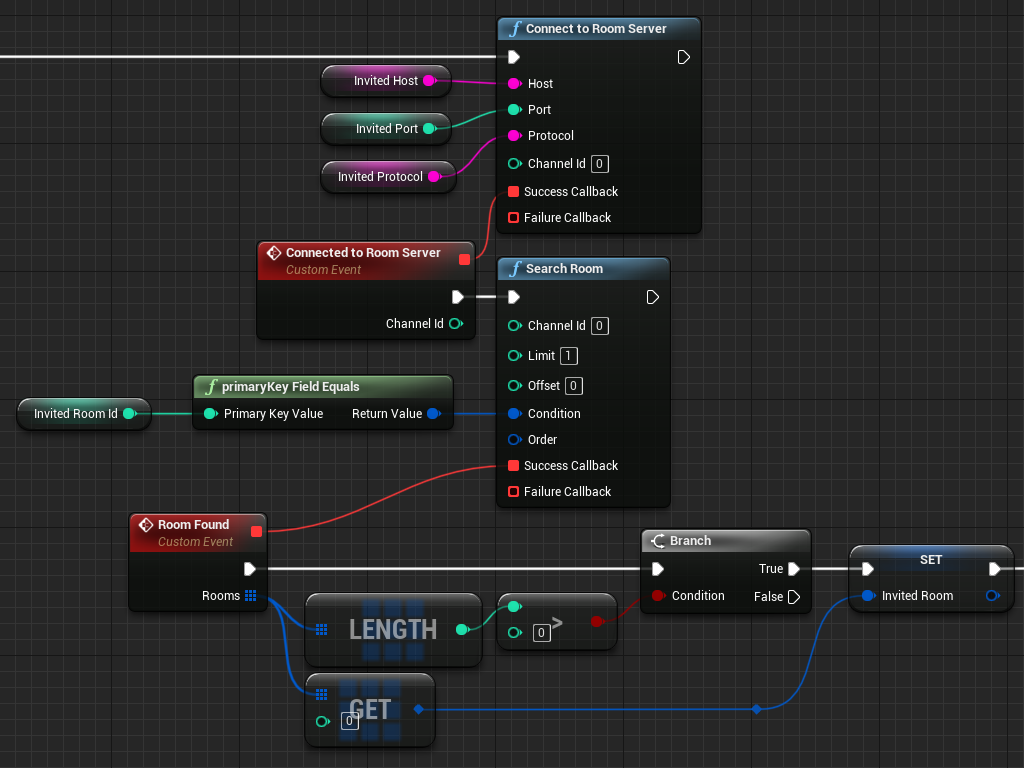
The Blueprint script above takes the variables
Invited Room Id
, Invited Host
, Invited Port
, and Invited Protocol
as inputs
and puts a Strix Room
struct in the variable Invited Room
when finished.
Finding a room server you are on¶
By the way, how you can know the address of the room server that you are currently on in a multiple room-server cluster, after creating a room by CreateNodeRoom for example?
It is not easy to find the information with a Blueprint script, and the following function in C++ does the job.
#include "CoreMinimal.h"
#include "Kismet/BlueprintFunctionLibrary.h"
#include "StrixBlueprintFunctionLibrary.h"
#include "MyBlueprintFunctionLibrary.generated.h"
UCLASS()
class MYPROJECT_API UMyBlueprintFunctionLibrary : public UBlueprintFunctionLibrary
{
GENERATED_BODY()
UFUNCTION(BlueprintCallable, Category = "MyBPLibrary", meta = (WorldContext = "WorldContextObject"))
static void GetCurrentNodeInfo(const UObject* WorldContextObject, int channelId,
FString& host, int32& port, FString& protocol);
};
#include "MyBlueprintFunctionLibrary.h"
#include <strix/net/channel/Protocol.h>
#include <strix/client/ingame/network/RoomServerConnection.h>
void UMyBlueprintFunctionLibrary::GetCurrentNodeInfo(const UObject* WorldContextObject,
int channelId,
FString& host, int32& port, FString& protocol)
{
UStrixNetworkFacade* strixNetworkFacade = UStrixNetworkFacade::GetInstance(WorldContextObject);
if (!strixNetworkFacade) return;
auto roomContext = strixNetworkFacade->GetRoomContextByChannelId(channelId);
if (!roomContext) return;
auto roomConnection = roomContext->GetConnection().lock();
if (!roomConnection) return;
host = FString(UTF8_TO_TCHAR(roomConnection->GetHost().c_str()));
port = roomConnection->GetPortNumber();
protocol = FString(strix::net::channel::ProtocolStringConverter::ToString(roomConnection->GetProtocol()).c_str());
}
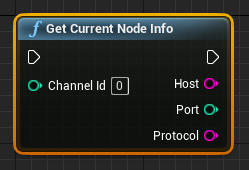
This Get Current Node Info function returns
the host name, port number, and protocol of the room server
of the room you are currently in on the specified Channel Id.
You can use this function as well as the standard Get Current Room
function
to know the quadruple that uniquely identifies your room
and is ready for inclusion in a party invitation.
Alternatively, you can run the script shown above using the quadruple to find the NodeRoom you are currently in and pass its primary key to friends.