Authentication/Authorization¶
Strix Cloud supports token authentication/authorization.
Strix Cloud’s game server can authenticate with other authentication servers using tokens without knowing important information such as user’s password.
You can use your own token, OAuth2, OpenID Connect, etc.
Sequence of Authentication/Authorization¶
The way authentication/authorization works is shown in the figure below. Authorization processing is performed by linking together the client application, Strix Cloud game server, and an external Web API server.
The Web API server must be prepared.
Prepare the following Web API as shown above.
Login API
Token Acquisition API
User Information Acquisition API
Login API¶
The login API is for authenticating over HTTPS communication. Any method can be used as the authentication method, so create a login process using your favorite method such as ID/password authentication or UUID authentication.
Token Acquisition API¶
The token acquisition API is an API for issuing an access token for Strix authentication. Check if you are logged in and return the token as a response.
Request
curl -X POST https://<Base URL>/api/access_token
Response
{
"access_token": "jERkHgIcAfe9xpSpqHUVxBuvLQTV77cO"
}
User Information Acquisition API¶
The User information acquisition API is an API that returns the user information associated with the token. This API is accessed to get user information from the game server side of the Strix Cloud.
This must be the POST request API. Also, the Authorization header contains an access token in the format of “Bearer <access token>”, so take out the access token part and return the user information associated with that access token in the response.
Request
curl -X POST -H 'Authorization: Bearer jERkHgIcAfe9xpSpqHUVxBuvLQTV77cO' https://<Base URL>/api/user
Response
{
"id": 123, // User id
"name": "Alice" // User name
}
User info should return an id and name.
The Strix Cloud Options Settings¶
First, enable the authentication/authorization option from the Strix Cloud options screen.
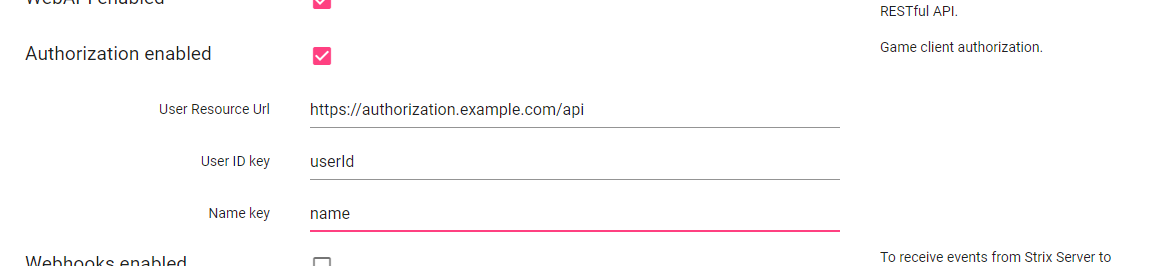
Then, prepare the Web API. Prepare an API that returns a token and an API that returns user information.
For the user resource acquisition API URL, specify the URL of the web server.
{
"id": 123,
"name": "Alice"
}
Call StrixNetwork.ConnectMasterServer
that sets the token in StrixNetwork.authorizationAccessToken
.
When using JoinRoom
, set the authentication URL in RoomJoinArgs.authUrl
authUrl
is included in the StrixNetwork.SearchRoom callback event and can be retrieved from roomInfo.nodeProperties["authUrl"]
string authUrl = "";
if (roomInfo.nodeProperties != null && roomInfo.nodeProperties.TryGetValue("authUrl", out object authUrlValue)) {
authUrl = authUrlValue.ToString();
}
RoomJoinArgs joinArgs = new RoomJoinArgs {
host = roomInfo.host,
port = roomInfo.port,
protocol = roomInfo.protocol,
roomId = roomInfo.roomId,
authUrl = authUrl,
memberProperties = memberProperties
};
StrixNetwork.instance.JoinRoom(joinArgs, handler, failureHandler, config);