ハンドラーの登録¶
注釈
以下に説明することは高度な機能です。プラグインのC++ソースコードを編集する必要があります。
Strixには現在、リレーメッセージ用の汎用ハンドラーがないため、カスタム実装で処理する必要があります。
以下に示すサンプル実装では、StrixBlueprintFunctionLibraryと、このライブラリが使用するStrixNetworkFacadeに新しい関数を2つ追加します。これらの関数は、ルームとダイレクトの各リレーメッセージのハンドラーとしてブループリント関数を登録するためのものです。
ほとんどの場合、このメソッドを変更する必要はありませんが、1つ注意すべき点があります。リレーメッセージの型が異なる可能性があるため、以下の最後の2つの関数のオブジェクト変換のステップを適切な型に変更する必要があります。
関数のセットアップ¶
// StrixBlueprintFunctionLibrary.h
/** Registers a handler to call when a Relay is received
* @param handler The callback to call when notification received
* @param channelId The channel to listen on
*/
UFUNCTION(BlueprintCallable, Category = "StrixBPLibrary", meta = (WorldContext = "WorldContextObject"))
static void RegisterStrixRoomRelayNotificationHandler(const UObject * WorldContextObject, const FStrixRoomRelayNotificationDelegate& handler, int32 channelId);
/** Registers a handler to call when a direct Relay is received
* @param handler The callback to call when notification received
* @param channelId The channel to listen on
*/
UFUNCTION(BlueprintCallable, Category = "StrixBPLibrary", meta = (WorldContext = "WorldContextObject"))
static void RegisterStrixDirectRelayNotificationHandler(const UObject * WorldContextObject, const FStrixDirectRelayNotificationDelegate& handler, int32 channelId);
// StrixBlueprintFunctionLibrary.cpp
void UStrixBlueprintFunctionLibrary::RegisterStrixRoomRelayNotificationHandler(const UObject * WorldContextObject, const FStrixRoomRelayNotificationDelegate& handler, int32 channelId)
{
// Get the network facade
UStrixNetworkFacade* strixNetwork = UStrixNetworkFacade::GetInstance(WorldContextObject);
// Check it exists
if (!strixNetwork)
{
UE_LOG(LogStrix, Log, TEXT("StrixBlueprintFunctionLibrary::RegisterStrixRoomRelayNotificationHandler - Strix network is not initialized."));
return;
}
// Call the register function
strixNetwork->RegisterRelayNotificationHandler(handler, channelId);
}
void UStrixBlueprintFunctionLibrary::RegisterStrixDirectRelayNotificationHandler(const UObject * WorldContextObject, const FStrixDirectRelayNotificationDelegate& handler, int32 channelId)
{
// Get the network facade
UStrixNetworkFacade* strixNetwork = UStrixNetworkFacade::GetInstance(WorldContextObject);
// Check it exists
if (!strixNetwork)
{
UE_LOG(LogStrix, Log, TEXT("StrixBlueprintFunctionLibrary::RegisterStrixRoomRelayNotificationHandler - Strix network is not initialized."));
return;
}
// Call the register function
strixNetwork->RegisterDirectRelayNotificationHandler(handler, channelId);
}
// StrixNetworkFacade.h
/** Registers a handler to call when a Relay is received
* @param handler The callback to call when notification received
* @param channelId The channel to listen on
*/
void RegisterRelayNotificationHandler(const FStrixRoomRelayNotificationDelegate& handler, int32 channelId);
/** Registers a handler to call when a direct Relay is received
* @param handler The callback to call when notification received
* @param channelId The channel to listen on
*/
void RegisterDirectRelayNotificationHandler(const FStrixDirectRelayNotificationDelegate& handler, int32 channelId);
ハンドラーの登録¶
// StrixNetworkFacade.cpp
// Register Relay handler
void UStrixNetworkFacade::RegisterRelayNotificationHandler(const FStrixRoomRelayNotificationDelegate& handler, int32 channelId)
{
// Get the room context for this channel. This represents the room connection on the client side.
auto context = m_roomContextsByChannelId.find(channelId);
if (context != m_roomContextsByChannelId.end())
{
// Get the match room client. This is what the handler will be added to.
auto matchRoomClient = context->second->GetMatchRoomClient();
if (matchRoomClient)
{
// Add the room Relay notification handler. This takes a lambda function to call when a message is received.
// The lambda itself takes a NotificationEventArgs<RoomRelayNotificationPtr> type which contains the message.
matchRoomClient->AddRoomRelayNotifiedHandler([=](strix::client::core::NotificationEventArgs<strix::client::room::message::RoomRelayNotificationPtr> args)
{
// Get the message that was sent.
auto message = args.m_data->GetMessageToRelay();
// In this case, the message contains an FString.
// The conversion is undertaken by the ConvertObjectToValue<FString> templated function.
FString messageText = strix::client::core::util::ObjectConverter::ConvertObjectToValue<FString>(message);
// Execute the handler.
// The handler is set in the Blueprint as a function.
handler.ExecuteIfBound(messageText);
});
}
}
}
void UStrixNetworkFacade::RegisterDirectRelayNotificationHandler(const FStrixDirectRelayNotificationDelegate& handler, int32 channelId)
{
// Get the room context for this channel. This represents the room connection on the client side.
auto context = m_roomContextsByChannelId.find(channelId);
if (context != m_roomContextsByChannelId.end())
{
// Get the match room client. This is what the handler will be added to.
auto matchRoomClient = context->second->GetMatchRoomClient();
if (matchRoomClient)
{
// Add the room Relay notification handler. This takes a lambda function to call when a message is received.
// The lambda itself takes a NotificationEventArgs<RoomDirectRelayNotificationPtr> type which contains the message.
matchRoomClient->AddRoomDirectRelayNotifiedHandler([=](strix::client::core::NotificationEventArgs<strix::client::room::message::RoomDirectRelayNotificationPtr> args)
{
// Get the message that was sent.
auto message = args.m_data->GetMessageToRelay();
// In this case, the message contains an FString.
// The conversion is undertaken by the ConvertObjectToValue<FString> templated function.
FString messageText = strix::client::core::util::ObjectConverter::ConvertObjectToValue<FString>(message);
// Execute the handler.
// The handler is set in the Blueprint as a function.
handler.ExecuteIfBound(messageText);
});
}
}
}
使用方法¶
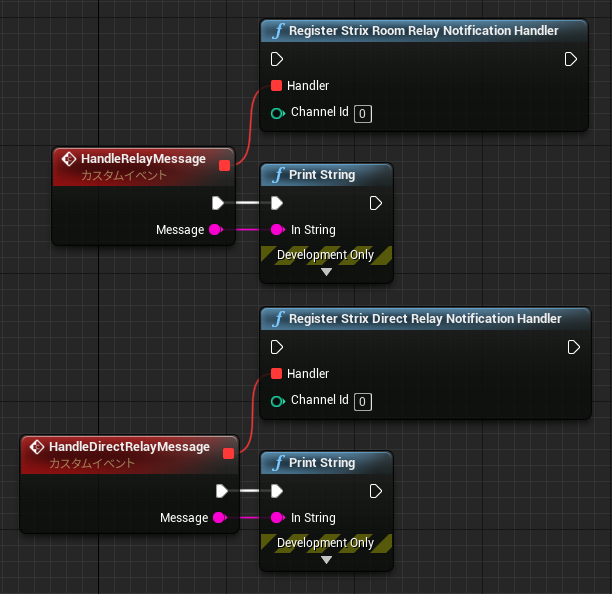